Project specification :
The project's objective was to create a C++ game engine in 4 weeks.
The graphical API used was either OpenGL or VulkanSDK. I chose to use Vulkan because, despite being more complex than OpenGL, this API is newer, and it is more likely that the industry will move in this direction. Vulkan also provides broader control over what happens in the computer.
The graphical API used was either OpenGL or VulkanSDK. I chose to use Vulkan because, despite being more complex than OpenGL, this API is newer, and it is more likely that the industry will move in this direction. Vulkan also provides broader control over what happens in the computer.
The specifications were as follows :
- Implement advanced and optimized graphics rendering.
- Create and manage GameObjects.
- Implement scene and 3D object management.
- Multi-platform support (Linux, Windows, etc.).
- Include development tools.
- Implement advanced and optimized graphics rendering.
- Create and manage GameObjects.
- Implement scene and 3D object management.
- Multi-platform support (Linux, Windows, etc.).
- Include development tools.
Pipeline Set-up :
The first, most hard and important step was to set-up the graphics pipeline for Vulkan.
Setting up the pipeline in a Vulkan project involves configuring the graphics pipeline, which is a key aspect of rendering in Vulkan. The graphics pipeline defines the stages through which data passes to be processed and rendered on the GPU.
Setting up the pipeline in Vulkan requires careful configuration of these stages to achieve the desired rendering behavior. Each step involves specifying how the GPU should process and render the input data.
The image on the right shows what processes the GPU has to go through to render the graphics.
The first, most hard and important step was to set-up the graphics pipeline for Vulkan.
Setting up the pipeline in a Vulkan project involves configuring the graphics pipeline, which is a key aspect of rendering in Vulkan. The graphics pipeline defines the stages through which data passes to be processed and rendered on the GPU.
Setting up the pipeline in Vulkan requires careful configuration of these stages to achieve the desired rendering behavior. Each step involves specifying how the GPU should process and render the input data.
The image on the right shows what processes the GPU has to go through to render the graphics.
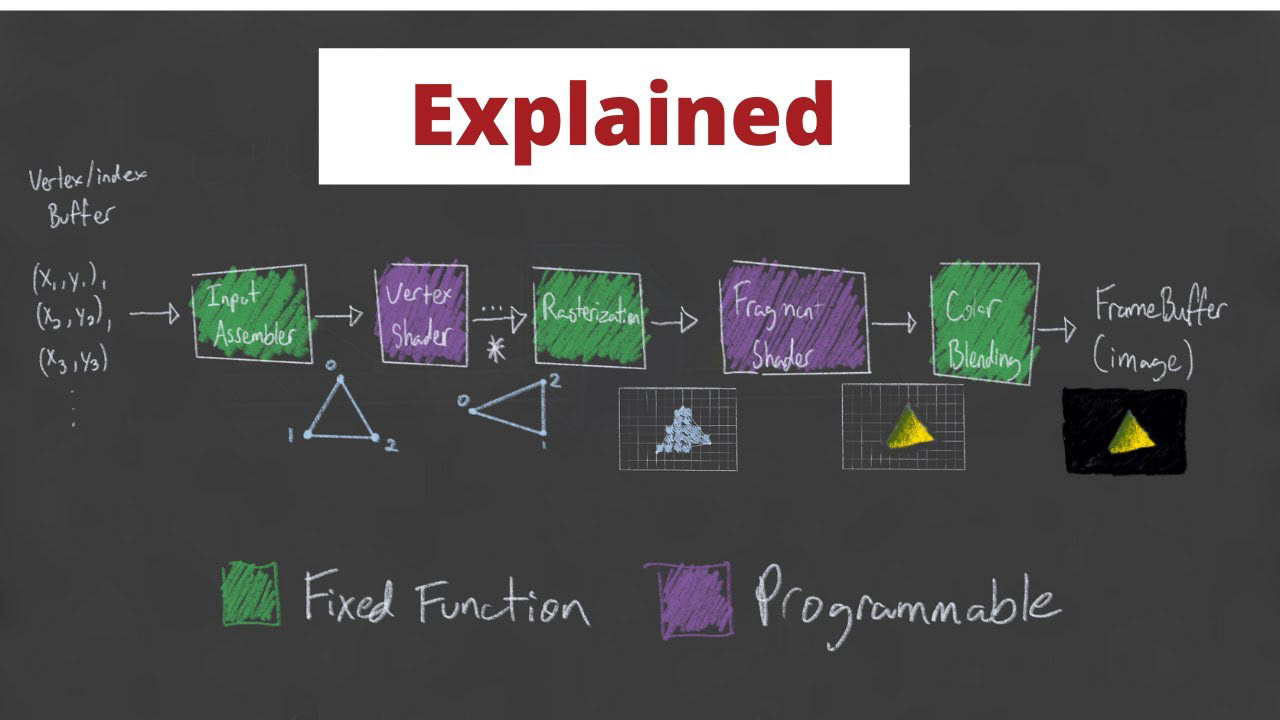
credit : @BrendanGalea
2D Rendering :
For this step, the focus was on rendering flat, two-dimensional objects using an orthographic projection. It involved preparing the necessary resources, configuring the graphics pipeline, and issuing drawing commands.
For 2D rendering, I used two shaders, one for the vertices and another one for the fragments.
For this step, the focus was on rendering flat, two-dimensional objects using an orthographic projection. It involved preparing the necessary resources, configuring the graphics pipeline, and issuing drawing commands.
For 2D rendering, I used two shaders, one for the vertices and another one for the fragments.
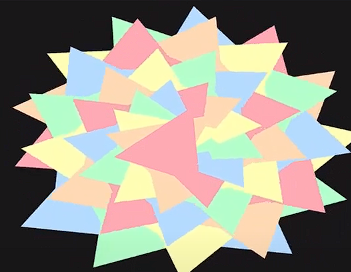
3D Rendering :
The focus here was on creating immersive three-dimensional scenes. This involves handling 3D models and setting up realistic projections. The steps include configuring the graphics pipeline, recording command buffers and presenting frames to achieve a visually compelling 3D experience in a Vulkan application.
Thanks to this step I learned how perspective and frustum works in games to set up a correct perspective camera.
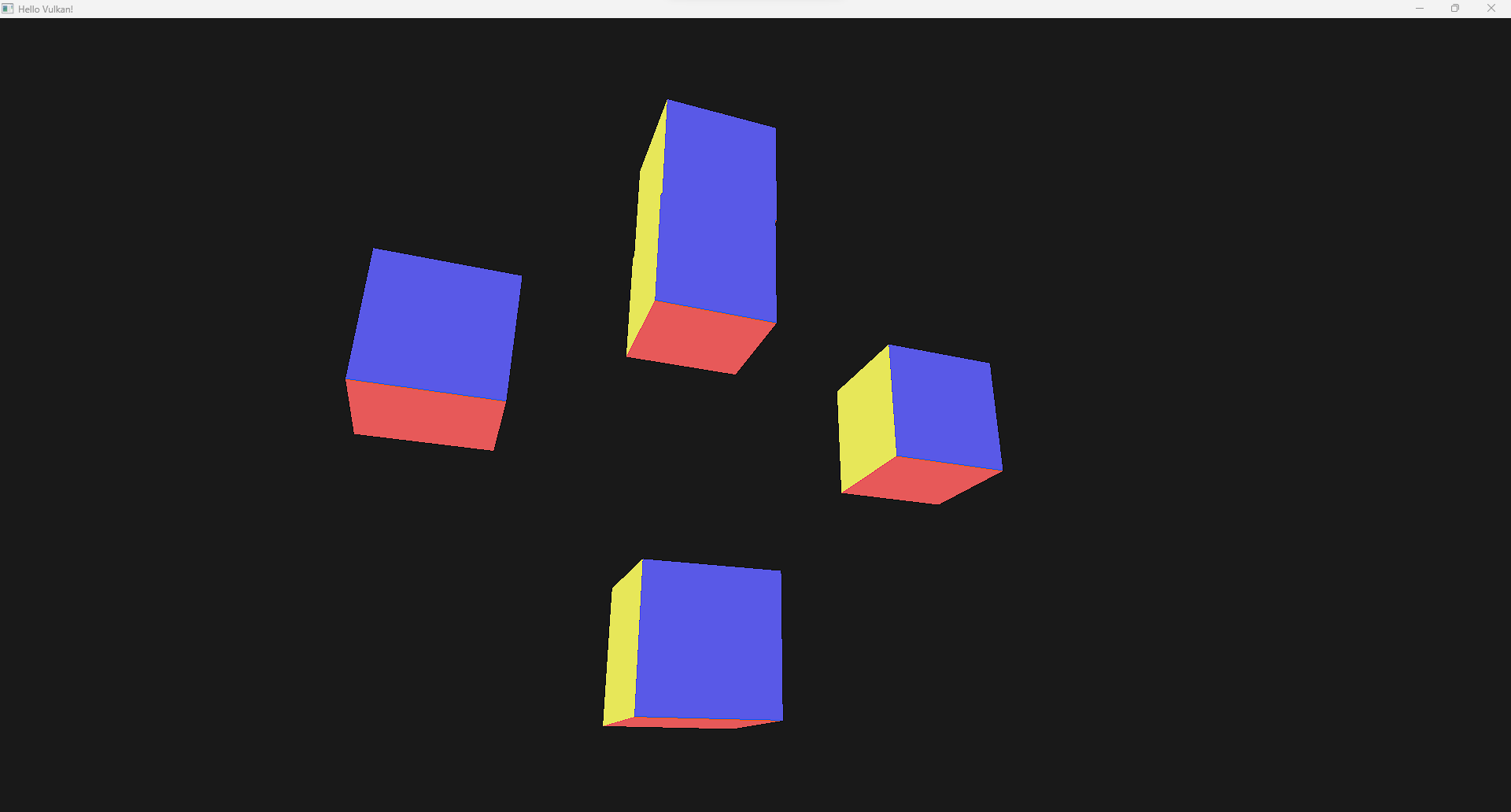
Transform, scale, rotation and collision :
The next step was to handle modifications for 3D objects.
By using matrixes and understanding how they works, we can apply transformations on the size, orientation or position of 3D models.
Additionaly, we implemented algorithms to detect collisions, using axis-aligned bounding boxes (AABB) and spherical hitboxes.
By using matrixes and understanding how they works, we can apply transformations on the size, orientation or position of 3D models.
Additionaly, we implemented algorithms to detect collisions, using axis-aligned bounding boxes (AABB) and spherical hitboxes.
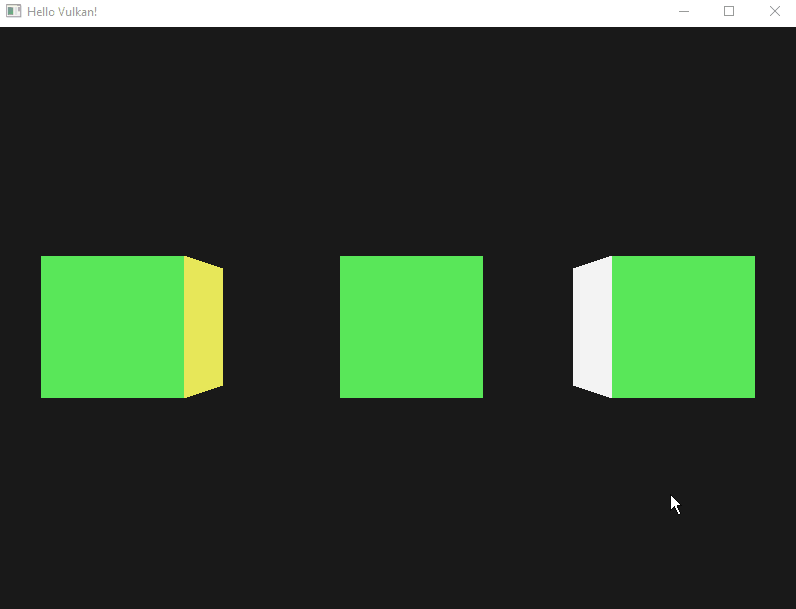
3D Models imports :
After setting up the transform component of 3D models, we added the possibility to import any obj file into our engine.
For this usage, we used the tinyobjloader library.
The challenge here was to scale the previous implementations to make them works properly with any 3D object.
Since Vulkan's coordinate system is not the usual one, obj files need to be exported with -Y for the up axis and Z for the forward axis.
For this usage, we used the tinyobjloader library.
The challenge here was to scale the previous implementations to make them works properly with any 3D object.
Since Vulkan's coordinate system is not the usual one, obj files need to be exported with -Y for the up axis and Z for the forward axis.
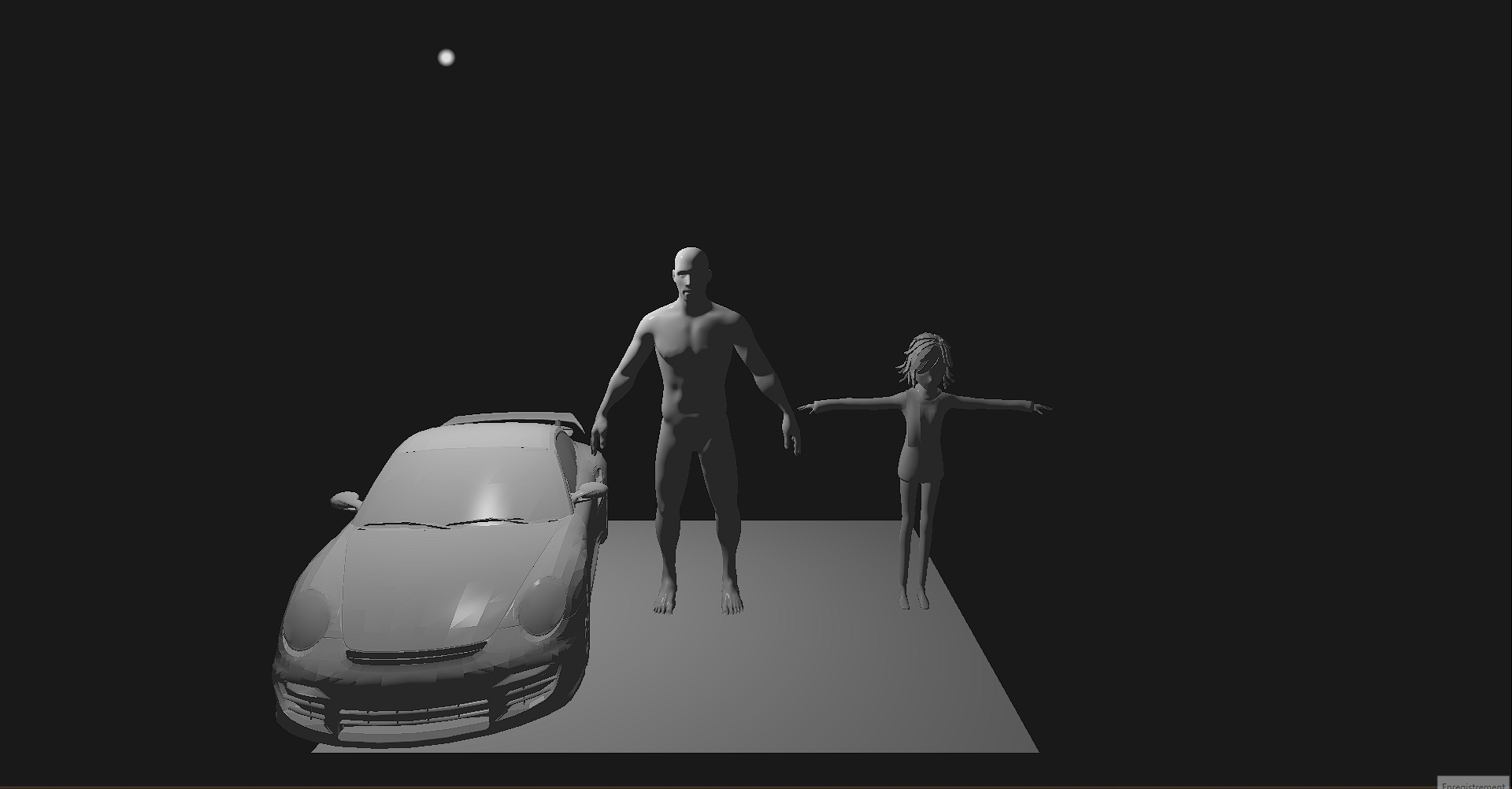
Lightning, alpha blending and transparency :
The next addition was directional lighting, which can simulate
the sun. This type of lighting uniformly illuminates anypoint touched by a light ray, with all rays considered parallel, as the light source is treated as infinitely distant from the hit point.
the sun. This type of lighting uniformly illuminates anypoint touched by a light ray, with all rays considered parallel, as the light source is treated as infinitely distant from the hit point.
Following that, "point lights" were introduced as smaller, non-parallel sources of light. These lights offer adjustable intensity and radius. We opted for realistic lighting calculations, limiting the number of lights but significantly improving graphic quality. Objects have customizable reflection intensity, allowing for effects like increased brightness on metallic surfaces.
Alpha blending and transparency functionalities were implemented to serve purposes, such as rendering glass or mirrors.
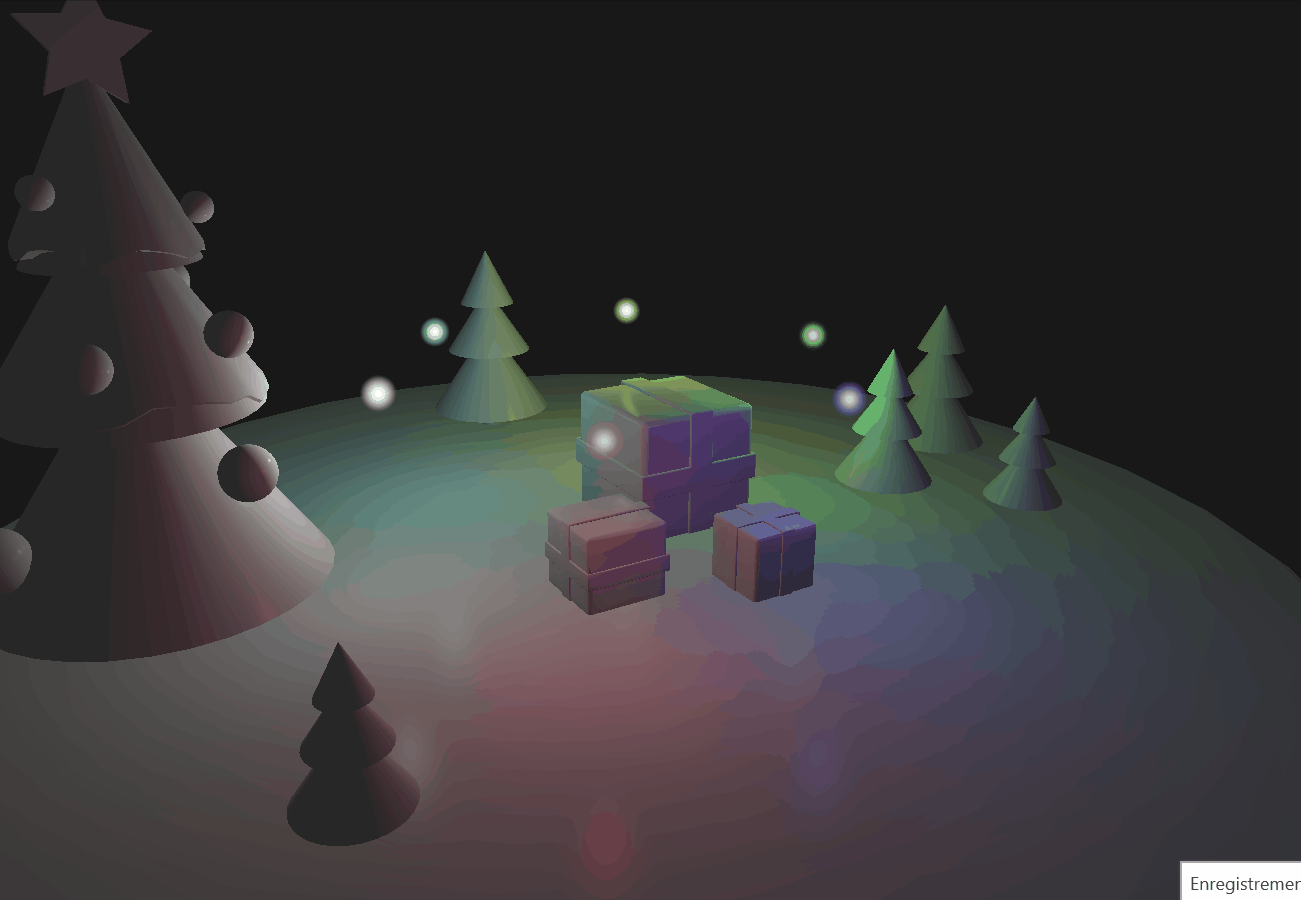
User Interface :
For the UI, we utilized the ImGui library. Drawing inspiration from Unity's UI, we crafted an inspector that showcases our object's components on the right and our hierarchy on the left.
The gif on the right demonstrates the UI implementation of the "Transform" component for a 3D object.
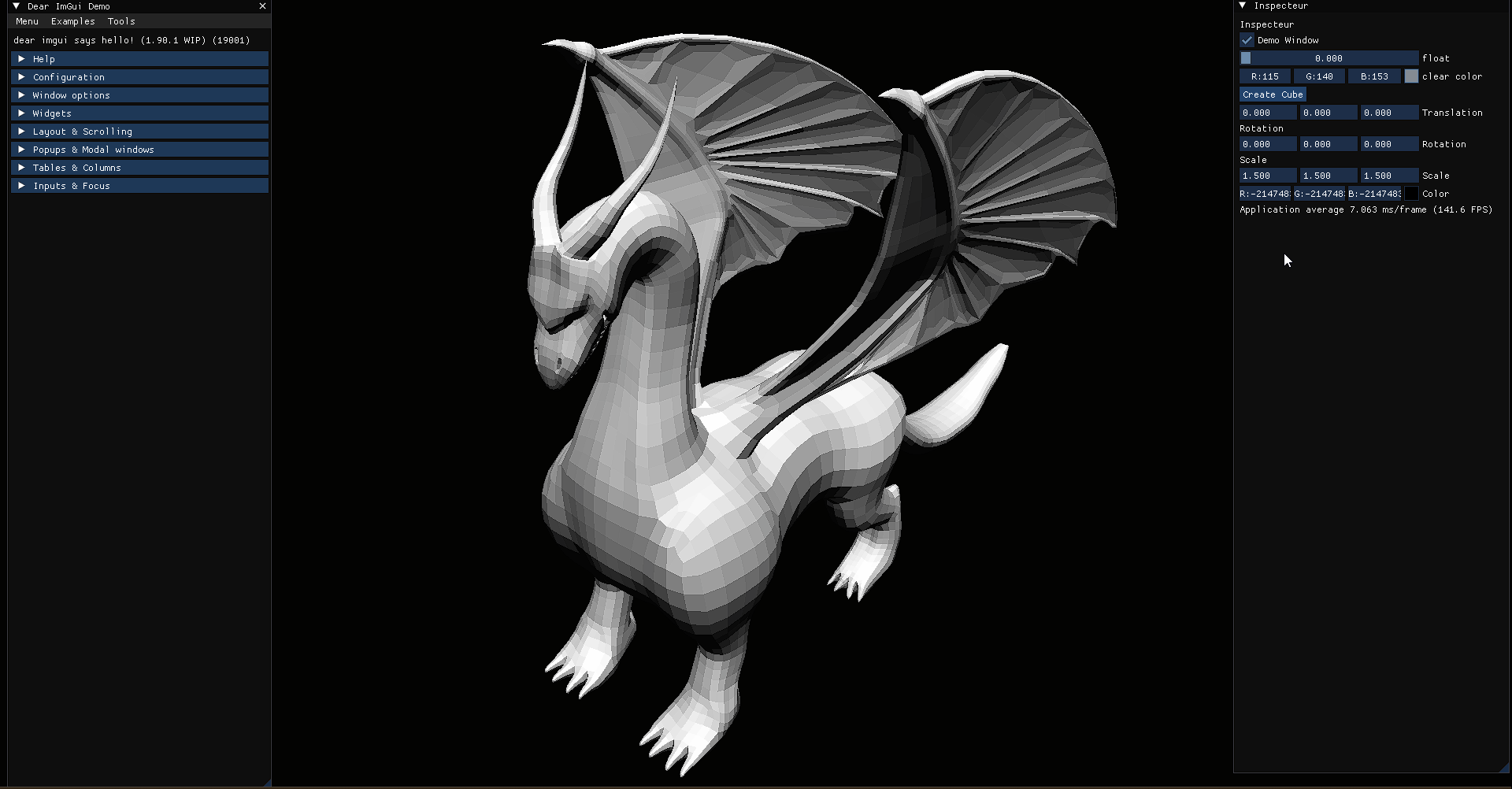
Gameplay Loop & User Inputs :
For the gameplay loop, we implemented two different approches. The first one is getting Inputs at every frame, it is similar to the Update() method from Unity. It is mainly used to collect inputs from the player as fast as possible.
The second one is handling Inputs at a fixed interval.
This approche is similar to the FixedUpdate() method from Unity. It is used for calculations that needs to be constant over time, for example acceleration.
The second one is handling Inputs at a fixed interval.
This approche is similar to the FixedUpdate() method from Unity. It is used for calculations that needs to be constant over time, for example acceleration.
Conclusion :
Before beginning this project, Vulkan was pretty initimidating because of its complexity. After learning the basics of Vulkan, I realised how graphics programming is working and I have a better understanding of how a GPU works. This project was pretty hard but I am proud that my team and I managed to overcome its difficulty and fullfill the specifications.
Possible improvements :
Possible improvements :
- Handling more than one texture.
- Handling more than one Scene.
- Add more components and customizable scripts.
Ressources used :
Ressources used :